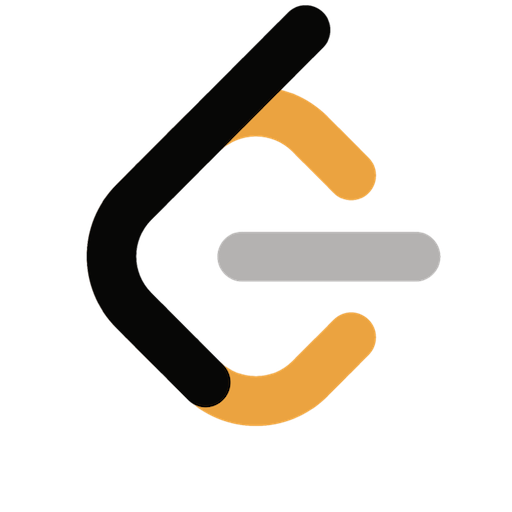
Leetcode - 495. Teemo Attacking
2022. 6. 13. 21:06
알고리즘
문제 설명 Input: timeSeries = [1,4], duration = 2 Output: 4 Explanation: Teemo's attacks on Ashe go as follows: - At second 1, Teemo attacks, and Ashe is poisoned for seconds 1 and 2. - At second 4, Teemo attacks, and Ashe is poisoned for seconds 4 and 5. Ashe is poisoned for seconds 1, 2, 4, and 5, which is 4 seconds in total. 배열 하나와 숫자 하나가 주어지는데 배열의 각 원소는 초(second), duration은 지속시간 정도로 생각하면 됩니다. 티모..
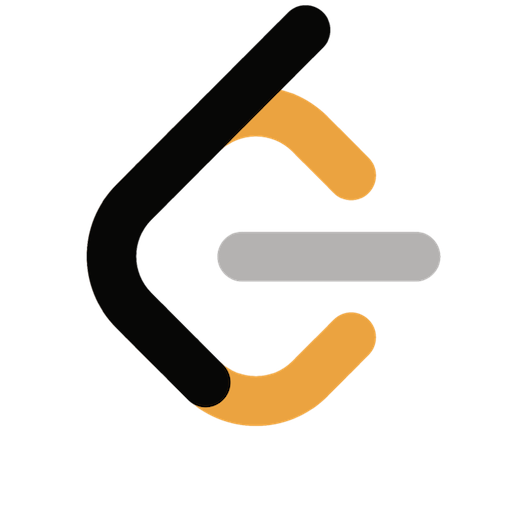
Leetcode - 1732. Find the Highest Altitude
2022. 6. 12. 18:16
알고리즘
문제 해설 Input: gain = [-5,1,5,0,-7] Output: 1 Explanation: The altitudes are [0,-5,-4,1,1,-6]. The highest is 1. 배열이 주어지는데 고도 0부터 시작하여 배열 원소만큼 위, 아래로 높아졌다가 낮아진다고 할 때 가장 높은 고도를 리턴하는 문제입니다 내가 푼 코드 const largestAltitude = function(gain) { let altitudes = 0 const sum = gain.reduce((prev, curr) => { if(prev > altitudes) altitudes = prev return prev + curr }, 0) return altitudes > sum ? altitudes : sum ..
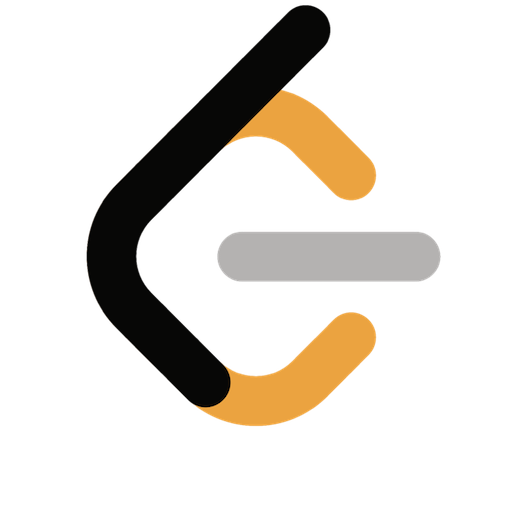
Leetcode - 1207. Unique Number of Occurrences
2022. 6. 11. 12:10
알고리즘
1. 문제 해설 Input: arr = [1,2,2,1,1,3] Output: true Explanation: The value 1 has 3 occurrences, 2 has 2 and 3 has 1. No two values have the same number of occurrences. Input으로 배열이 주어지는데 주어지는 배열에 각 원소 개수(위 예시에서 1의 개수는 3개, 2의 개수는 2개, 3의 개수는 1개) 들이 중복되지 않는다면 true 중복된다면 false를 반환하는게 문제입니다. 2. 내가 푼 코드 const uniqueOccurrences = function(arr) { const uniqueMap = new Map(); for(let i = 0; i
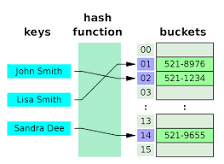
Javascript Map object
2022. 6. 9. 22:45
알고리즘
1. Hash Table이란 알고리즘을 공부하면서 자료구조도 같이 알아볼 때 자주 나오는 Hash가 있습니다 Hash는 간단하게 생각한다면 배열에서 index대신 key로 접근하는 방법이라고 생각하면 됩니다 key로 들어오는 값을 hashing(해싱)이라고 해서 실제 주소로 변경시키는 작업을 거쳐 저장시키게 되며 이렇게 저장하게 된다면 별다른 참조없이 특정 값에 바로 접근할 수 있다는 장점을 가지고 있습니다 배열을 생각해보면 [0,1,2,3] 배열이 있다고 할 때 index(2)의 값을 가져오려고 0번부터 접근하지만 만약 hash 구조를 사용한다면 0번부터 찾지않고 해싱 방식에 따라 key를 주소로 변환하여 바로 접근하게 됩니다 2. Javascript에서 hash table JS에서 지원하는 해시테이블..
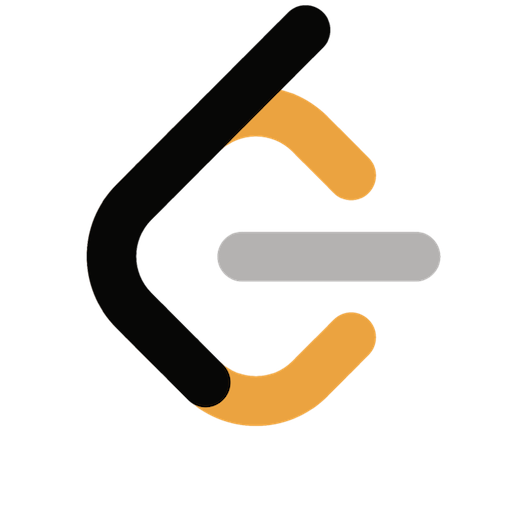
Leetcode - 1773. Count Items Matching a Rule
2022. 6. 8. 22:36
알고리즘
문제 items 배열이 주어질 때 ruleValue와 일치하는 원소의 갯수를 찾는 문제였습니다. 이때 ruleKey도 같이 주어지는데 단순히 ruleValue가 안에 있는지를 확인하는게 아닌 items 배열 원소를 item이라고 할 때 item의 각 자리마다 대응되는 ruleKey를 통해 해당 인덱스의 value가 ruleValue인지 찾는 문제였습니다 예제 Input: items = [["phone","blue","pixel"],["computer","silver","lenovo"],["phone","gold","iphone"]], ruleKey = "color", ruleValue = "silver" Output: 1 Explanation: There is only one item matching t..
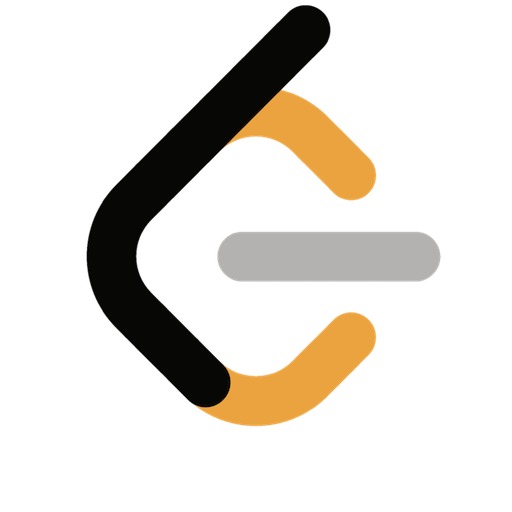
Leetcode - How Many Numbers Are Smaller Than the Current Number
2022. 6. 7. 21:38
알고리즘
Input: nums = [8,1,2,2,3] Output: [4,0,1,1,3] Explanation: For nums[0]=8 there exist four smaller numbers than it (1, 2, 2 and 3). For nums[1]=1 does not exist any smaller number than it. For nums[2]=2 there exist one smaller number than it (1). For nums[3]=2 there exist one smaller number than it (1). For nums[4]=3 there exist three smaller numbers than it (1, 2 and 2). 배열 nums에서 각 원소보다 더 작은 원소..
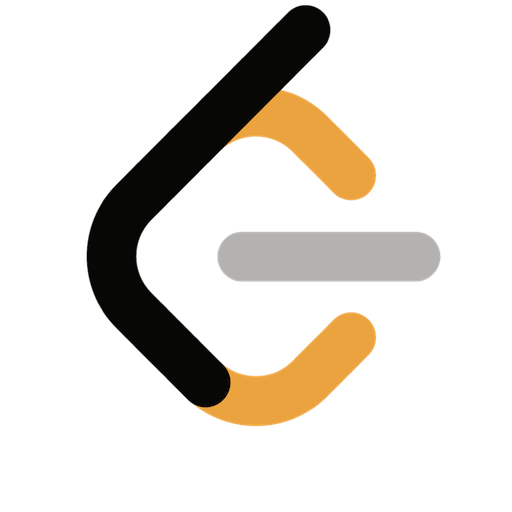
Leetcode - Palindrome Number
2022. 6. 7. 21:20
알고리즘
Palindrome Number 정수 x가 주어질 때 x가 회문 숫자인지 구하는 것 문자열로 바꿨을 때 맨앞과 맨뒤부터 시작하여 한칸씩 이동하며 같은 정수인지 확인하는 것으로 해결하였습니다. 이때 종료되는 경우를 생각했을 때 앞에서 시작하는 번호와 뒤에서 시작하는 번호가 같거나, 앞에서 시작하는 번호가 뒤보다 더 클때를 종료해야할 때로 생각했고 그 외 경우는 반복문을 계속 돌려 진행하였습니다 해결한 코드 var isPalindrome = function(x) { const NumberToString = x + "" let front = 0 let back = NumberToString.length-1 while(front