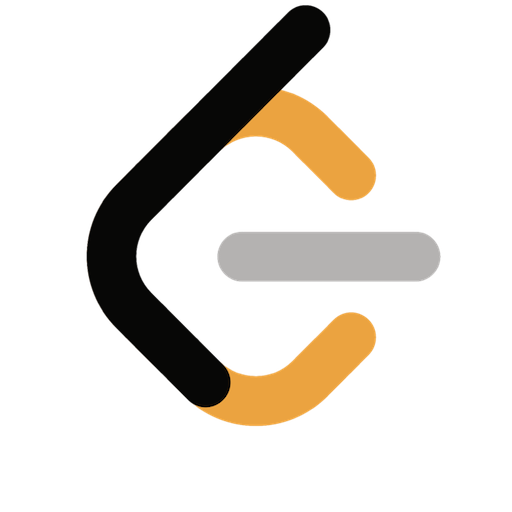
문제 설명
Input: timeSeries = [1,4], duration = 2
Output: 4
Explanation: Teemo's attacks on Ashe go as follows:
- At second 1, Teemo attacks, and Ashe is poisoned for seconds 1 and 2.
- At second 4, Teemo attacks, and Ashe is poisoned for seconds 4 and 5.
Ashe is poisoned for seconds 1, 2, 4, and 5, which is 4 seconds in total.
배열 하나와 숫자 하나가 주어지는데 배열의 각 원소는 초(second), duration은 지속시간 정도로 생각하면 됩니다.
티모가 주어진 배열 원소 시간에 공격을 하게되면 duration만큼 독에 걸리게 되며 위 예시에서 1초, 4초에 지속시간만큼의 독을 걸게 되고 총 독에 걸린 시간만큼을 return하면 되는 문제입니다.
이때 1초에 독을 걸고 2초에 독을걸게되면 지속시간은 갱신되어 다시 지속시간만큼 값이 올라가게 됩니다.
내가 푼 코드
const findPoisonedDuration = function(timeSeries, duration) {
let result = 0;
for(let i = 0; i < timeSeries.length; i++) {
if(!timeSeries[i+1]) {
result += duration
break;
}
const durationRefresh = timeSeries[i+1] - timeSeries[i];
if(durationRefresh >= duration) result+= duration
else result += durationRefresh
}
return result
};
경우를 두가지로 나눴는데 우선 지속시간보다 텀이 큰 경우, 텀보다 지속시간이 큰 경우로 나누었고 만약 지속시간보다 텀이 크다면 지속시간을, 텀보다 지속시간이 크다면 텀을 더해주는 방식으로 해결하였습니다.
다만 범위가 매우 커서 효율적이진 못했습니다
남이 푼 코드
c++로 푼 코드를 확인했지만 코드 자체는 어렵지않아 쉽게 이해가 되었습니다.
제가 푼 코드같은 경우에는 if로 조건을 여러 개를 걸어놔 가독성측면에서도 많이 떨어졌지만 이 코드 같은 경우에는 결국에 두 가지 중 최솟값만 더하면 되는 경우임과 동시에 맨 마지막 배열은 반복문을 거치지 않아도 되기 때문에 훨씬 깔끔하고 효율적인 코드라고 생각하였습니다
int findPoisonedDuration(vector<int>& timeSeries, int duration) {
const int n = timeSeries.size();
int ans = 0;
for (int i = 0; i < n - 1; i++) {
ans += min(duration, timeSeries[i+1] - timeSeries[i]);
}
return ans + duration;
}
'알고리즘' 카테고리의 다른 글
자주 나오는 정렬 알고리즘 (0) | 2022.10.24 |
---|---|
Leetcode - 807. Max Increase to Keep City Skyline (0) | 2022.06.17 |
Leetcode - 1732. Find the Highest Altitude (0) | 2022.06.12 |
Leetcode - 1207. Unique Number of Occurrences (0) | 2022.06.11 |
Javascript Map object (0) | 2022.06.09 |