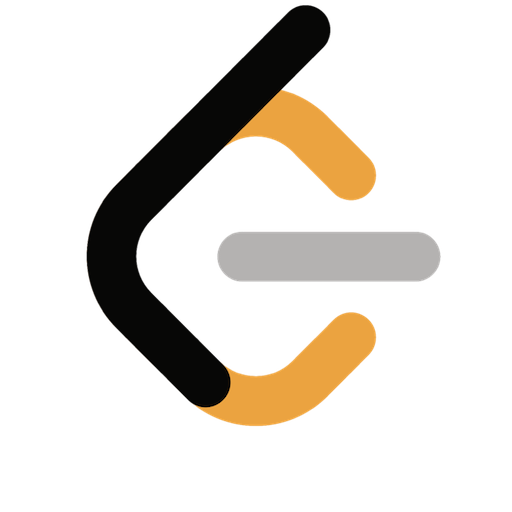
Input: nums = [8,1,2,2,3]
Output: [4,0,1,1,3]
Explanation:
For nums[0]=8 there exist four smaller numbers than it (1, 2, 2 and 3).
For nums[1]=1 does not exist any smaller number than it.
For nums[2]=2 there exist one smaller number than it (1).
For nums[3]=2 there exist one smaller number than it (1).
For nums[4]=3 there exist three smaller numbers than it (1, 2 and 2).
배열 nums에서 각 원소보다 더 작은 원소의 갯수를 세는 문제였습니다
해결한 코드
브루트 포스 알고리즘을 통해 단순히 이중 for문으로 돌려 해결할 수 있을거 같았고 배열을 return해야 하니 map을 사용해서 만들어주었습니다
const smallerNumbersThanCurrent = function(nums) {
const result = nums.map(v => {
let count = 0
for(let i = 0; i < nums.length; i++) {
if(v > nums\[i\])
count++
}
return count
})
return result
};
이후 참고한 코드
다른 코드들과 비슷하게 풀었다고 생각되서 많이 찾아보진 않았지만 잘 이해되지 않는 코드가 있어서 추가적으로 찾아볼 예정입니다
var smallerNumbersThanCurrent = function(nums) {
const ranks = [...nums].sort((a, b) => b - a).reduce((res, n, i) => {
res[n] = nums.length - 1 - i;
return res;
}, {});
return nums.map(n => ranks[n]);
};
'알고리즘' 카테고리의 다른 글
Leetcode - 1732. Find the Highest Altitude (0) | 2022.06.12 |
---|---|
Leetcode - 1207. Unique Number of Occurrences (0) | 2022.06.11 |
Javascript Map object (0) | 2022.06.09 |
Leetcode - 1773. Count Items Matching a Rule (0) | 2022.06.08 |
Leetcode - Palindrome Number (0) | 2022.06.07 |