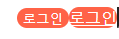
https://styled-components.com/
styled-components
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress 💅🏾
styled-components.com
npm install --save styled-components
기본적인 문법들
import styled from "styled-components";
const Father = styled.div`
display: flex;
justify-content: center;
align-items: center;
`;
return (
<Father>
</Father>
);
기본적인 styled-components의 사용법은 이렇습니다
const 컴포넌트명 = styled.html태그;
import styled from "styled-components";
const Father = styled.div`
display: flex;
justify-content: center;
align-items: center;
`;
const BoxTwo = styled.div`
background-color : red;
width:100px;
height:100px;
`;
const BoxOne = styled.div`
background-color : green;
width:100px;
height:100px;
`;
return (
<Father>
<BoxTwo/>
<BoxOne/>
</Father>
);
이렇게 선언한다면 red박스와 green박스 하나씩 w100 h100크기로 Father안에서 수평으로 만들어 질 것입니다.
하지만 위와 같은 방식은 코드가 중복되니 깔끔하지 않아 위와 같은 문제를 해결하기 위해 props로 색깔을 전달해 줄 수 있고 중복을 줄일 수 있습니다.
import styled from "styled-components";
const Father = styled.div`
display: flex;
justify-content: center;
align-items: center;
`;
const Box = styled.div`
background-color : ${(props)=> props.bgColor};
width:100px;
height:100px;
`;
return (
<Father>
<Box bgColor="red"/>
<Box bgColor="green"/>
</Father>
);
이렇게 props로 전달해준다면 위와 같은 결과가 나오지만 코드를 상당히 줄일 수 있습니다.
또 여기서 의문이 생길 수 있습니다 만약에 새로운 컴포넌트를 만들고 싶은데 이 구조가 Box 컴포넌트와 비슷할 때 즉, 기존 컴포넌트에 있는 모든 CSS 속성을 가져와서 새로운 것들을 추가하고 싶을 때
const Box = styled.div`
background-color : ${(props)=> props.bgColor};
width:100px;
height:100px;
`;
const Circle = styled.div`
background-color : ${(props)=> props.bgColor};
width:100px;
height:100px;
border-radius: 50%;
`;
여기서 border-radius만 추가 되었는데 또 중복이 생겨버렸다
const Box = styled.div`
background-color : ${(props)=> props.bgColor};
width:100px;
height:100px;
`;
const Circle = styled(Box)`
border-radius: 50%;
`;
하지만 이렇게 쓴다면 Box에 있는 CSS 속성들을 가져오면서 중복을 없애고 새로운 코드를 추가할 수 있다.
const Box = styled(같은 값을 가지는 컴포넌트)
클래스의 개념으로 생각해봤을 때 상속받는 다는 것을 알 수 있습니다.
HTML 태그와 속성
const Btn = styled.button`
color:white;
background-color: tomato;
border: 0;
border-radius: 15px;
`;
return (
<Father>
<Btn>로그인</Btn>
<Btn as ="a" href="/">로그인</Btn>
</Father>
);
아까와 같은 구조에서 같은 CSS 속성을 가지는데 HTML태그만 바꾸고자 할 때는 “as”를 사용한다 위와 같이 사용한다면 Btn 이름으로 버튼 태그를 정의 하였지만 컴포넌트에서 as를 붙여 a 태그로 만들어 줄 수 가 있다
다음으로는 HTML태그에 속성이 필요할 수가 있다 예를 들면 required처럼 입력을 꼭 받아야 한다던지 value가 필요하던지 등등 기존 문법에서 attrs를 추가한 뒤 속성을 전달하면 된다. 하지만 코드의 가독성을 고려해봐야하기 때문에 상황에 따라서 사용할 필요가 있습니다
const Input = styled.input.attrs({required: true, minLength: 10, value:"americano"})`
background-color: #e64327;
`;
return (
<Father>
<Input/>
<Input/>
<Input/>
<Input/>
<Input/>
</Father>
);
Animation
const animation = keyframes`
0% {
transform: rotate(0deg);
border-radius: 0px;
}
50% {
border-radius: 100px;
}
100% {
transform: rotate(360deg);
border-radius: 0px;
}
`;
CSS처럼 @keyframes로 정의하면 된다 @만 때고 다른 것들과 비슷하게 CSS구문을 사용하면 됩니다
const Box = styled.div`
height: 100px;
width: 100px;
background-color: tomato;
display: flex;
align-items: center;
justify-content: center;
animation: ${animation} 1s linear infinite;
`;
animation: ${animation} 1s linear infinite; 템플릿 리터럴, 즉 백틱이기 때문에 ${}만 주의하면 됩니다
하위태그 selector
SCSS를 사용할 때와 비슷하다
https://www.notion.so/SCSS-SASS-dff2f1614ba34130bf8be1b0ba275e37
const Box = styled.div`
height: 100px;
width: 100px;
background-color: tomato;
display: flex;
align-items: center;
justify-content: center;
animation: ${animation} 1s linear infinite;
span { //box안에 있을때만 사용할 수 있다.
&:hover {
font-size: 98px;
}
}
`;
위 코드를 보면 Box안에 하위태그가 그대로 들어가게 되고 &를 사용하여 부모연산자를 생략하기도 한다 &:hover를 풀어쓰면 span:hover 가 된다. 하지만 span:hover 를 쓰게되면 span 안에다 쓸 수 없으니 가독성있게 &:hover를 사용하는게 좋습니다.
그 외에도 Box안에 다른 컴포넌트의 스타일을 불러올 수 있는데 아래와 같이 사용할 수 있습니다
아래와 같이 사용한다면 Box에 있는 Emoji만 hover했을 때 달라질 수 있다고 이해하면 됩니다
const Box = styled.div`
height: 100px;
width: 100px;
background-color: tomato;
display: flex;
align-items: center;
justify-content: center;
animation: ${animation} 1s linear infinite;
${Emoji} { //box안에 있을때만 사용할 수 있다.
&:hover {
font-size: 98px;
}
}
`;
const Emoji = styled.span`
font-size: 36px;
`
- Darkmode(Theme)
styled component는 dark모드를 상당히 쉽게 사용할 수 있도록 지원을 해주고 있습니다. styled component에서 색상을 미리 지정해놓고 바꾸기 쉽기 때문입니다
import React from 'react';
import ReactDOM from 'react-dom';
import {ThemeProvider} from "styled-components"
import App from './App';
const darkTheme = {
textColor: "whitesmoke",
backgroundColor : "#111"
}
const lightTheme = {
textColor: "#111",
backgroundColor : "whitesmoke"
}
ReactDOM.render(
<React.StrictMode>
<ThemeProvider theme={darkTheme}>
<App />
</ThemeProvider>
</React.StrictMode>,
document.getElementById('root')
);
ThemeProvider 로 다크모드를 상당히 쉽게 만들어 주는데 darkTheme일때의 색깔과 lightTheme일때의 백그라운드와 텍스트 컬러를 지정하고 Theme으로 전달해주게 되면 됩니다.
이후 저는 상태관리 라이브러리로 관리로 관리하였습니다
( 규모가 클수록 세세한 부분까지 많은 컬러를 지정해준다고 합니다)
<React.StrictMode>
<ThemeProvider theme={darkTheme}>
<App />
</ThemeProvider>
</React.StrictMode>,
document.getElementById('root')
'TIL > 개념정리' 카테고리의 다른 글
Framer-motion 간단한 사용법 정리 (0) | 2022.07.16 |
---|---|
@keyframes - animation 사용법 (0) | 2022.07.14 |
File upload 취약점 (0) | 2022.07.12 |
CSRF와 방어 방법이 무엇인지 (0) | 2022.07.09 |
XSS - 크로스 사이트 스크립팅 (0) | 2022.07.05 |